Loading... # C# 使用RabbitMQ的完整图解 > 之前的文章一直在csdn上面放着,今天转过来 > https://blog.csdn.net/qq_40732336/article/details/113186732 ## 1.前言 Message Queue消息队列,简称MQ,是一种应用程序对应用程序的通信方法,应用程序通过读写出入队列的消息来通信,而无需专用连接来链接它们。消息传递指的是程序之间通过在消息中发送数据进行通信,而不是通过直接调用彼此通信。 MQ是消费-生产者模型的一个典型代表,一端往消息队列中不断写入消息,而另一端则可以读取或者订阅队列中的消息。 ## 2. 安装 > 提示:以下的链接下载特别慢,建议到我的网盘下载,包括案例,速度特快(回复`rabbit`) ### 1. 下载RabbitMQ [RabbitMQ服务](http://www.rabbitmq.com/download.html):http://www.rabbitmq.com/download.html  安装完RabbitMQ服务后,会在Windows服务中看到。如果没有Erlang运行环境,在安装过程中会提醒先安装Erlang环境 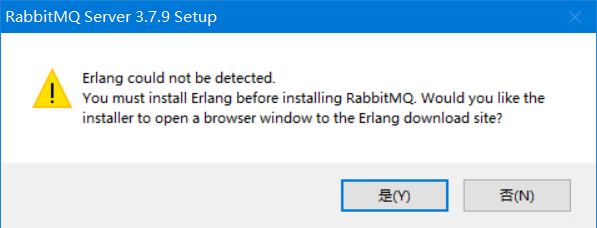 ### 2. OTP 点击是就会直接跳转,或者[点击链接](http://www.erlang.org/downloads)进行下载 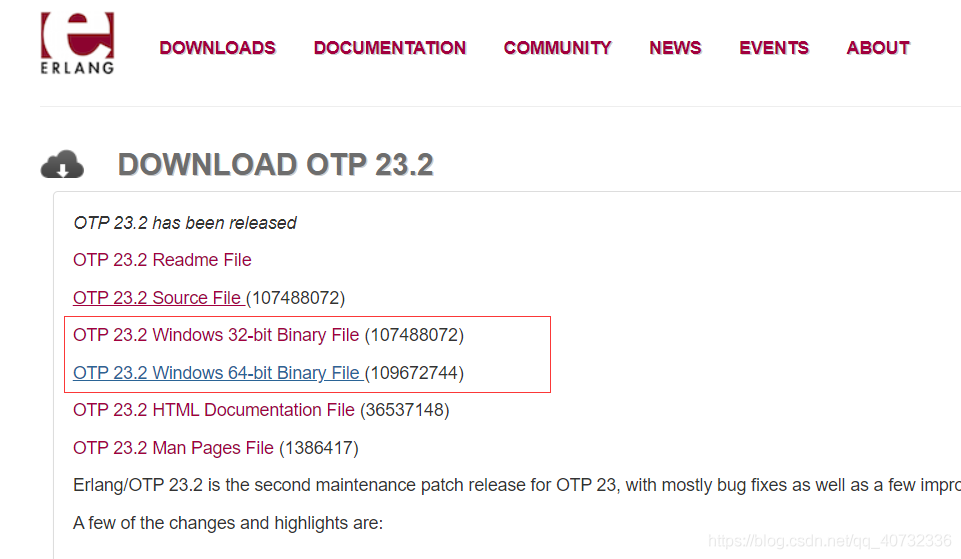 下载完后进行暗安装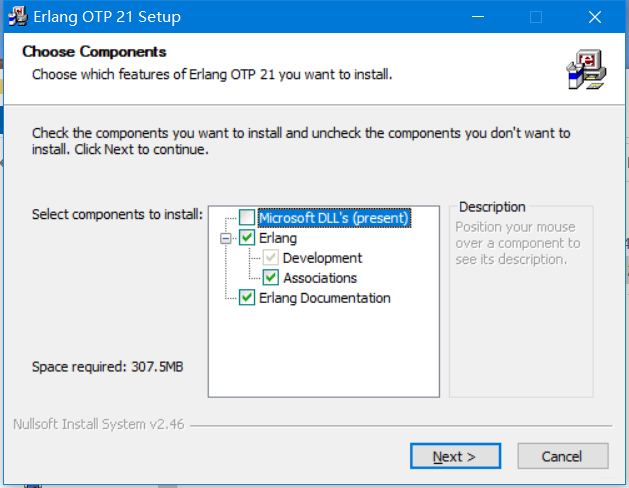 ## 3.使用 打不开`web管理工具`的请看参考 > web管理工具的地址是:`http://localhost:15672` > 初始用户名:`guest` > 初始密码:`guest` ## 4.代码实现 #### 消息生产者 ``` class Program { static void Main(string[] args) { try { ConnectionFactory factory = new ConnectionFactory(); factory.HostName = Constants.MqHost; factory.Port = Constants.MqPort; factory.UserName = Constants.MqUserName; factory.Password = Constants.MqPwd; using (IConnection conn = factory.CreateConnection()) { using (IModel channel = conn.CreateModel()) { //在MQ上定义一个持久化队列,如果名称相同不会重复创建 channel.QueueDeclare("MyFirstQueue", true, false, false, null); while (true) { string customStr = Console.ReadLine(); RequestMsg requestMsg = new RequestMsg(); requestMsg.Name = string.Format("Name_{0}", customStr); requestMsg.Code = string.Format("Code_{0}", customStr); string jsonStr = JsonConvert.SerializeObject(requestMsg); byte[] bytes = Encoding.UTF8.GetBytes(jsonStr); //设置消息持久化 IBasicProperties properties = channel.CreateBasicProperties(); properties.DeliveryMode = 2; channel.BasicPublish("", "MyFirstQueue", properties, bytes); //channel.BasicPublish("", "MyFirstQueue", null, bytes); Console.WriteLine("消息已发送:" + requestMsg.ToString()); } } } } catch (Exception e1) { Console.WriteLine(e1.ToString()); } Console.ReadLine(); } } ``` #### 消息消费者 ``` class Program { static void Main(string[] args) { try { ConnectionFactory factory = new ConnectionFactory(); factory.HostName = Constants.MqHost; factory.Port = Constants.MqPort; factory.UserName = Constants.MqUserName; factory.Password = Constants.MqPwd; using (IConnection conn = factory.CreateConnection()) { using (IModel channel = conn.CreateModel()) { //在MQ上定义一个持久化队列,如果名称相同不会重复创建 channel.QueueDeclare("MyFirstQueue", true, false, false, null); //输入1,那如果接收一个消息,但是没有应答,则客户端不会收到下一个消息 channel.BasicQos(0, 1, false); Console.WriteLine("Listening..."); //在队列上定义一个消费者 QueueingBasicConsumer consumer = new QueueingBasicConsumer(channel); //消费队列,并设置应答模式为程序主动应答 channel.BasicConsume("MyFirstQueue", false, consumer); while (true) { //阻塞函数,获取队列中的消息 BasicDeliverEventArgs ea = (BasicDeliverEventArgs)consumer.Queue.Dequeue(); byte[] bytes = ea.Body; string str = Encoding.UTF8.GetString(bytes); RequestMsg msg = JsonConvert.DeserializeObject<RequestMsg>(str); Console.WriteLine("HandleMsg:" + msg.ToString()); //回复确认 channel.BasicAck(ea.DeliveryTag, false); } } } } catch (Exception e1) { Console.WriteLine(e1.ToString()); } Console.ReadLine(); } } ``` ## 5.总结 最后的运行结果 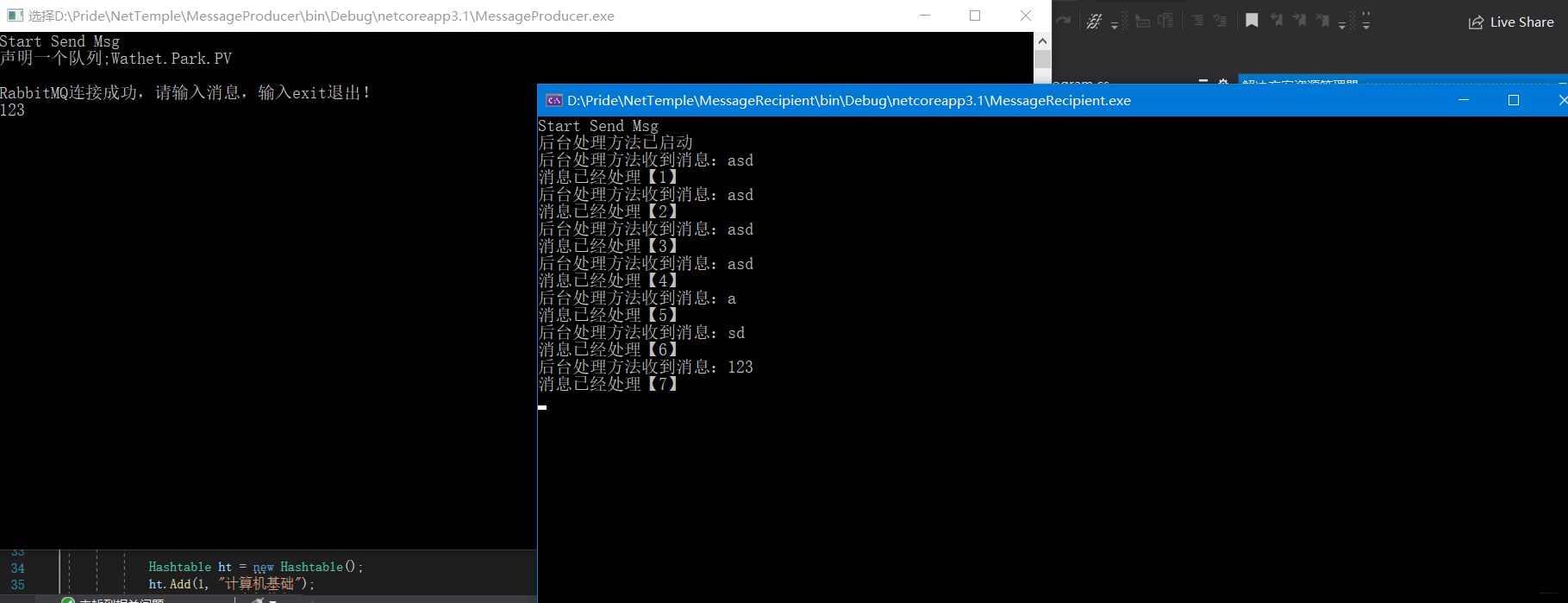 [消息生产者_代码GITHUB](https://github.com/PrideJoy/NetTemple/blob/master/MessageProducer/Program.cs) `https://github.com/PrideJoy/NetTemple/blob/master/MessageProducer/Program.cs` 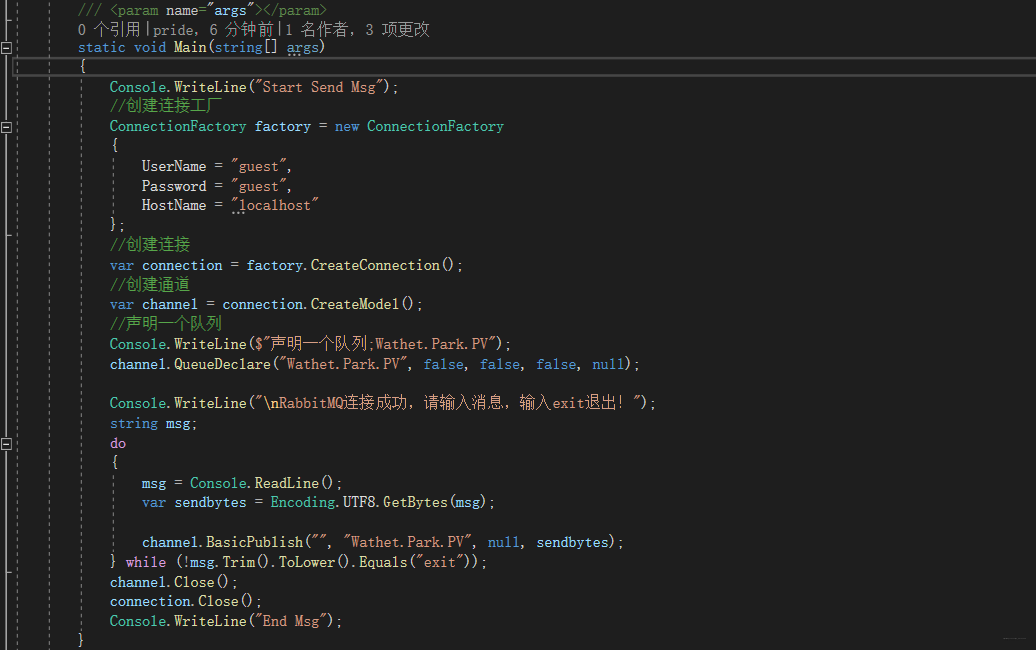 [消息消费者 _代码GITHUB](https://github.com/PrideJoy/NetTemple/blob/master/MessageRecipient/Program.cs) 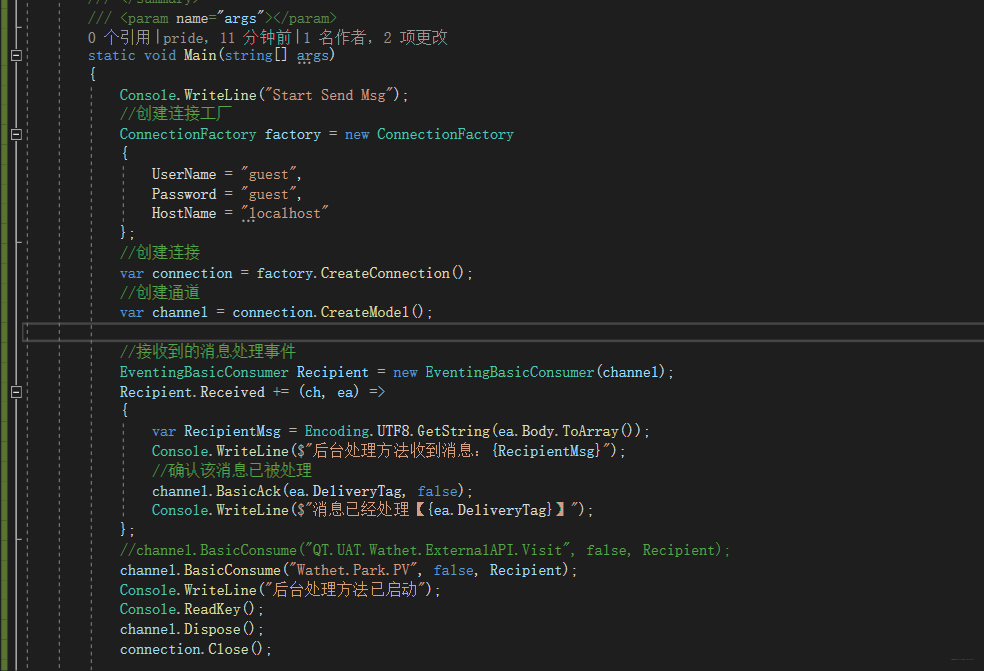 ## 6.项目说明 (微信公众号)分享最新的Net和Core相关技术以及实战技巧,更重要的是分享Net项目,不容错过的还有书籍,手写笔记等等。 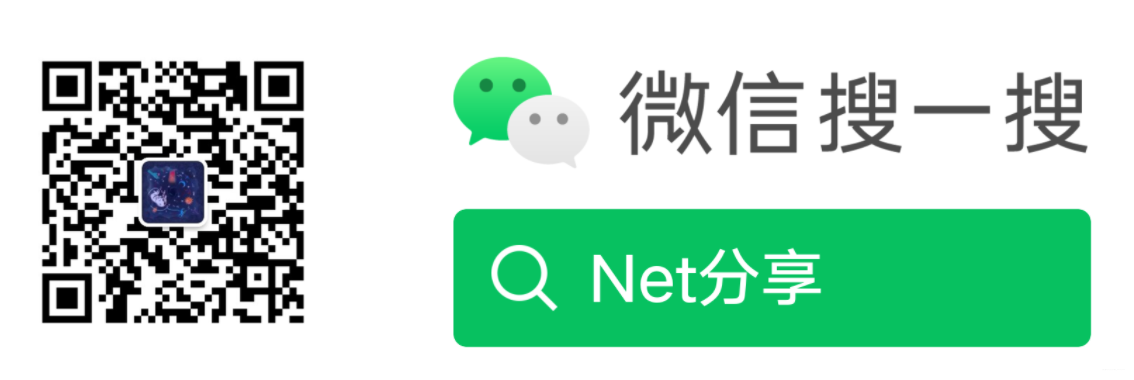 ## 参考 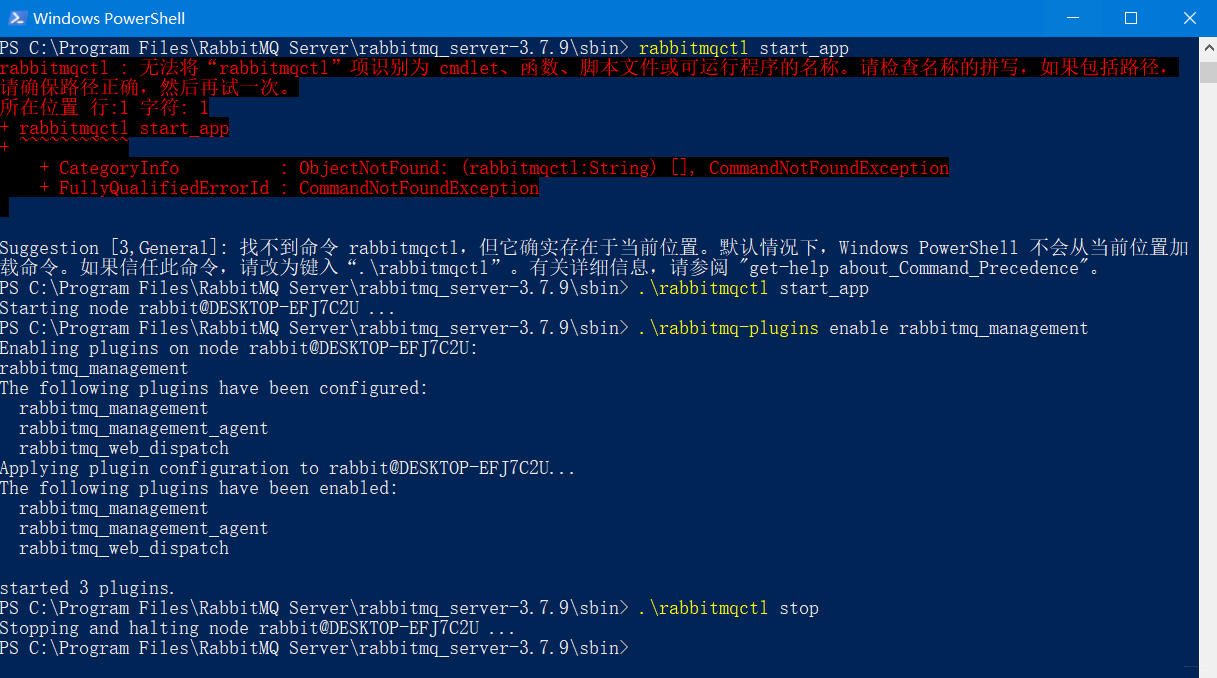 [1.安装RabbitMQ无法访问(http://localhost:15672)_解决方案](https://www.jianshu.com/p/e041f5ed10c7) [2.C#调用RabbitMQ实现消息队列](https://www.cnblogs.com/mq0036/p/11726733.html) [3.在C#中使用消息队列RabbitMQ](https://www.jb51.net/article/122378.htm) [4.rabbitmq 安装过程中遇到的 rabbitmq-plugins不是内部命令或外部命令](https://blog.csdn.net/weixin_43997764/article/details/104848466) [5.win10 安装RabbitMQ 报错无法将“rabbitmq-plugins”项识别为 cmdlet、函数、脚本文件或可运行程序的名称](https://blog.csdn.net/zhangxiaojun211/article/details/100368642) 最后修改:2022 年 02 月 25 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请随意赞赏